Programming in C#
Corso
A Milano
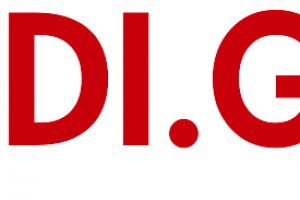
Hai bisogno di un coach per la formazione?
Ti aiuterà a confrontare vari corsi e trovare l'offerta formativa più conveniente.
Descrizione
-
Tipologia
Corso
-
Luogo
Milano
-
Inizio
Scegli data
Overview of Writing Applications using C# Datatypes, Operators, and Expressions C# Programming Language Constructs Implementing Edit Functionality for the Students List Implementing Insert Functionality for the Students List
Sedi e date
Luogo
Inizio del corso
Inizio del corso
Opinioni
Materie
- Web master
- .net framework
- C
- C#
- Framework
- C++
Programma
Implementing Delete Functionality for the Students List
Displaying the Student Age
After completing this module, students will be able to:
Describe the architecture of .NET Framework applications and use the features that Visual Studio 2012 and C# provide to support .NET Framework development.
Use the basic data types, operators, and expressions provided by C#.
Use standard C# programming constructs.
This module explains how to create and call methods, catch and handle exceptions. This module also describes the monitoring requirements of large-scale applications.
Creating and Invoking Methods
Creating Overloaded Methods and Using Optional and Output Parameters
Handling Exceptions
Monitoring Applications
Refactoring the Enrolment Code
Validating Student Information
Saving Changes to the Class List
After completing this module, students will be able to:
Create and invoke methods, pass parameters to methods, and return values from methods.
Create overloaded methods, and use optional parameters and output parameters.
Catch and handle exceptions and write information to the event log.
Explain the requirement for implementing logging, tracing, and profiling when building large-scale applications.
This module describes how to implement the basic structure and essential elements of a typical desktop application, including using structures and enumerations, collections, and events.
Implementing Structs and Enums
Organizing Data into Collections
Handling Events
Adding Navigation Logic to the Application
Creating Data Types to Store User and Grade Information
Displaying User and Grade Information
After completing this module, students will be able to:
Define and use structures and enumerations.
Create and use simple collections for storing data in-memory.
Create, subscribe to, and raise events.
This module explains how to create classes, define and implement interfaces, and create and use generic collections. This module also describes the differences between value types and reference types in C#.
Creating Classes
Defining and Implementing Interfaces
Implementing Type-safe Collections
Implementing the Teacher, Student, and Grade Types as Classes
Adding Data Validation to the Grade Class
Displaying Students in Name Order
Enabling Teachers to Modify Class and Grade Data
After completing this module, students will be able to:
Create and use custom classes.
Define and implement custom interfaces.
Use generics to implement type-safe collections.
This module explains how to use inheritance to create a class hierarchy and extend a .NET Framework class. This module also describes how to create generic classes and define extension methods.
Creating Class Hierarchies
Extending .NET Framework Classes
Creating Generic Types
Creating and Inheriting from the User Base Class
Implementing Password Complexity by Using an Abstract Method
Creating the ClassFullException Class
After completing this module, students will be able to:
Define abstract classes and inherit from base classes to create a class hierarchy.
Inherit from .NET Framework classes and use extension methods to add custom functionality to the inherited class.
Create generic classes and methods.
This module explains how to read and write data by using file input/output (I/O) and streams, and how to serialize and deserialize data in different formats.
Reading and Writing Files
Serializing and Deserializing Data
Performing I/O Using Streams
Serializing the Data for the Grades Report as XML
Previewing the Grades Report
Persisting the Serialized Grades Data to a File
After completing this module, students will be able to:
Read and write data to and from the file system by using file I/O.
Convert data into a format that can be written to or read from a file or other data source.
Use streams to send and receive data to or from a file or other data source.
This module explains how to create and use an entity data model for accessing a database, and how to use LINQ to query and update data.
Creating and Using Entity Data Models
Querying Data by Using LINQ
Updating Data by Using LINQ
Creating an Entity Model from the The School of Fine Arts Database
Updating Student and Grade Data Using the Entity Framework
Extending the Entity Model to Validate Data
After completing this module, students will be able to:
Create an entity data model, describe the key classes contained in the model, and customize the generated code.
Use LINQ to query and work with data.
Use LINQ to insert, update, and delete data.
This module explains how to use the types in the System.Net namespace, and WCF Data Services, to query and modify remote data.
Accessing Data Across the Web
Accessing Data in the Cloud
Creating a WCF Data Service for the SchoolGrades Database
Integrating the WCF Data Service into the Application
Retrieving Student Photographs Over the Web (if time permits)
After completing this module, students will be able to:
Use the classes in the System.Net namespace to send and receive data across the Web.
Create and use a WCF Data Service to access data in the cloud.
This module explains how to build and style a graphical user interface by using XAML. This module also describes how to display data in a user interface by using data binding.
Using XAML to Design a User Interface
Binding Controls to Data
Styling a User Interface
Customizing the Appearance of Student Photographs
Styling the Logon View
Animating the StudentPhoto Control (If Time Permits)
After completing this module, students will be able to:
Define XAML views and controls to design a simple graphical user interface.
Use XAML data binding techniques to bind XAML elements to a data source and display data.
Add styling and dynamic transformations to a XAML user interface.
This module explains how to improve the throughput and response time of applications by using tasks and asynchronous operations.
Implementing Multitasking by using Tasks and Lambda Expressions
Performing Operations Asynchronously
Synchronizing Concurrent Access to Data
Ensuring that the User Interface Remains Responsive When Retrieving Data for Teachers
Providing Visual Feedback During Long-Running Operations
After completing this module, students will be able to:
Create tasks and lambda expressions to implement multitasking.
Define and use asynchronous methods to improve application responsiveness.
Coordinate concurrent access to data shared across multiple tasks by using synchronous primitives and concurrent collections.
This module explains how to integrate unmanaged libraries and dynamic components into a C# application. This module also describes how to control the lifetime of unmanaged resources.
Creating and Using Dynamic Objects
Managing the Lifetime of Objects and Controlling Unmanaged Resources
Generating the Grades Report by Using Microsoft Office Word
Controlling the Lifetime of Word Objects by Implementing the Dispose Pattern
After completing this module, students will be able to:
Integrate unmanaged code into a C# application by using the Dynamic Language Runtime.
Control the lifetime of unmanaged resources and ensure that they are disposed properly.
This module explains how to examine the metadata of types by using reflection, create and use custom attributes, generate managed code at runtime, and manage different versions of assemblies.
Examining Object Metadata
Creating and Using Custom Attributes
Generating Managed Code
Versioning, Signing and Deploying Assemblies
Creating the IncludeInReport Attribute
Generating the Report
Storing the Grades.Utilities Assembly Centrally
After completing this module, students will be able to:
Examine the metadata of objects at runtime by using reflection.
Create and use custom attribute class.
Generate managed code at runtime by using CodeDOM.
Manage different versions of an assembly and deploy an assembly to the Global Assembly Cache.
This module explains how to encrypt and decrypt data by using symmetric and asymmetric encryption.
Implementing Symmetric Encryption
Implementing Asymmetric Encryption
Encrypting the Grades Report
Decrypting the Grades Report
After completing this module, students will be able to:
Perform symmetric encryption by using the classes in the System.Security namespace.
Perform asymmetric encryption by using the classes in the System.Security namespace.
Hai bisogno di un coach per la formazione?
Ti aiuterà a confrontare vari corsi e trovare l'offerta formativa più conveniente.
Programming in C#